webpackを使ってJSとCSSをコンパイルする(ES6 / Sass)
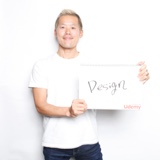
Railsでいきなりwebpack使おうとしたけど、基本がわかってなさすぎたので、webpack単体で遊んでみることにした。GulpとかGruntとかあるけど、個人的にはwebpackが一番分かりやすい。
いままでちょっとしたコーディングならCodekit使ってたけど、webpackでもいいかな。
とりあえず node.js と npm を最新にしておこうか。
shell
1
2
3
4
5
6
7
8
9
10
$ npm update -g npm
# nodeのインストールには n がいいよ
$ npm install -g n
# これで最新の安定版が入る
$ n stable
$ n
# => 複数入ってたら選べる
webpackイントール
1
$ npm install webpack -g
とりあえず、webpackの感じをつかむためコマンドを実行してみる。
下のファイルをつくる。
entry.js
javascript
1
document.write("It works.");
で、実行すると bundle.js
が生成される。
shell
1
$ webpack ./entry.js bundle.js
それをHTMLで読み込んでいる。
大雑把に言うとこの流れがすべて。
html
1
2
3
4
5
6
7
8
<html>
<head>
<meta charset="utf-8">
</head>
<body>
<script type="text/javascript" src="bundle.js" charset="utf-8"></script>
</body>
</html>
bundle.js は生成されたJS。
entry.js は依存関係が全部入っているJS。
外部JSをバンドルしてみる
JS Bundler と言われるくらいだからね。
content.js
javascript
1
module.exports = "This is content.js!";
entry.js
javascript
1
document.write(require("./content.js"));
コンパイルすると、HTMLに This is content.js! と出てるはず。
shell
1
$ webpack ./entry.js bundle.js
CSSだってバンドルできる
なんと便利な。
JS以外を読み込むには Loader と呼ばれるものが必要。
インストール。
shell
1
$ npm install css-loader style-loader
CSSを書く。
css
1
2
3
4
body {
background: red;
fort-size: 2.0em;
}
読み込む。
entry.js
javascript
1
2
require("!style!css!./style.css");
document.write(require("./content.js"));
Javascript にCSSの読み込みを書くという変な感じ。
!style!css!./style.css
なので注意。
!style!css! と毎回書くのはイケてないので、configに書いてしまおうというのが下に続く。
Configをつくる
webpack.config.js
javascript
1
2
3
4
5
6
7
8
9
10
11
12
module.exports = {
entry: "./entry.js",
output: {
path: __dirname,
filename: "bundle.js"
},
module: {
loaders: [
{ test: /\.css$/, loader: "style!css" }
]
}
};
これがあれば webpack
だけでいい。
shell
1
$ webpack
ファイルへの変更を監視する
これでOK。
shell
1
$ webpack --watch
なんならサーバーを立ち上げることもできる。
インストールが必要。
shell
1
$ npm install webpack-dev-server -g
こんな感じで起動。
shell
1
$ webpack-dev-server
http://localhost:8080/webpack-dev-server/index.html に行くと確認できるはず。
Sassもコンパイルしたい
CSSだけではツライ。Sassも欲しい。
shell
1
$ npm install sass-loader --save-dev
webpack.config.js に追加する。
javascript
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
module.exports = {
entry: "./entry.js",
output: {
path: __dirname,
filename: "bundle.js",
},
module: {
loaders: [
{ test: /\.css$/, loader: "style!css" },
{
test: /\.sass$/,
loaders: ["style", "css", "sass"]
}
]
}
};
サーバー使ってたら、config変更した時点で再起動した方がいいかも。
loaderの変更が反映されなくてハマった。
ES6も書きたい
せっかくなのでCoffeeScriptでなくてES6にしてみよう。
shell
1
$ npm install babel-loader babel-core babel-preset-es2015 --save-dev
webpack.config.jsはこんな感じになった。
javascript
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
module.exports = {
entry: "./entry.js",
output: {
path: __dirname,
filename: "bundle.js",
},
module: {
loaders: [
{ test: /\.css$/, loader: "style!css" },
{
test: /\.sass$/,
loaders: ["style", "css", "sass"]
},
{
test: /\.js?$/,
exclude: /(node_modules|bower_components)/,
loader: 'babel',
query: {
cacheDirectory: true
}
}
]
}
};
exclude に node_modules いれないと、nodeモジュールのJS全部見ていくので遅くなる。
やたらファイル多いもんね…。
サーバー再起動して、OKなはず!
以上。
追記
vagrant で --watchコマンド使っても動かなかった。
原因が分からないので、 ホスト側(Mac)から wabpack --watch
コマンドを叩くことにした。
ちゃんとコンパイルされるので問題ないが vagrant の webpack で最終的にビルドするので、できたら vagrant 上のものを使いたい。
だれかやり方教えて…
参考
https://webpack.github.io/docs/usage.html
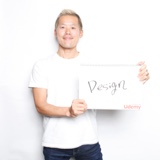