Ruby cheat sheet / variable / class / module
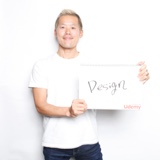
It's just a cheat sheet for Ruby beginners.
ruby
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
# Module is a part which is shared by many classes
# You can include module like, 'include Keyboard'
# It's neseccery to put module before class definition
module Keyboard
# You can access instance variable if it exist
def type(key)
puts "You typed [#{key}] with #{self.name}.\n\n"
end
end
class Phone
# Module
include Keyboard
# Class constant
TYPE = "mobile"
# Class variable
# This is shared by every Phone instance
# This can't be called by accessor method
@@count = 0
# Class instance variable
@camera = "NO"
# Execute when Phone new instance is created
# Same as constructor in JAVA
def initialize(name, version, color)
# Instance variable
# This is unique in each instance
@name = name
@version = version
@color = color
# Change class variable when instance is created
@@count += 1
end
# Accessor method
# You can access instance variable like, phone.name
# These have different permission
attr_reader :name # read
attr_writer :version # write
attr_accessor :color # read/write
def start
puts "Welcome to #{self.color} #{self.name}! (instance method)\n\n"
end
def sleep(wake_up_time)
puts "See you at #{wake_up_time}, zzz.... (instance method)\n\n"
end
def exit
puts "Good bye! from #{self.name} (instance method)\n\n"
end
def change_color(color)
# Change value of instance variable
self.color = color
puts "You changed color to #{color} (instance method)\n\n"
end
# This is class method, using 'self.'
def self.show_device_count
puts "@@count : #{@@count} ('Class' method with class variable)\n\n"
end
# This is class method, using 'self.'
def self.camera?
puts "Camera : #{@camera}\n\n"
end
end
class SmartPhone < Phone
# Module
# include Keyboard
# Additional method only for SmartPhone
def take_photo
puts "You can take photo with smartphone!\n\n"
# Private function
store_photo(self)
end
# Override method and execute super class method inside
def change_color(color, surface)
super(color)
puts "Now your phone's surface is polished! (Override instance method)\n\n"
end
# This method doesn't work
# @camera is not accessible from SmartPhone class
def self.camera?
puts "Camera : #{@camera} (of course dosn't work)\n\n"
end
# You can call private method within SmartPhone class
private
def store_photo(device)
puts " -> Photo is saved in #{device.name}. (Private method)\n\n"
end
end
# Create new instance from Phone class
# The arguments are used for initialize method
phone = Phone.new('Traditional Phone', 1.0, 'black')
# Class constant
puts "\n\nTYPE : #{Phone::TYPE}"
# Class method with class instance variable
puts Phone.camera?
# Instance method
phone.start
# Instance methods with arguments
phone.change_color('white')
# You can write version but can't read
# because of accessor method
phone.version = 1.1
now = Time.now()
wake_up_time = now + 2*60*60
phone.sleep(wake_up_time)
# Method from Keyboard module
phone.type('t')
# Class method
Phone.show_device_count
# Create instance from SmartPhone class
iphone = SmartPhone.new('iPhone', 5.2, 'black')
iphone.start
# Now you can see class variable is changed
Phone.show_device_count
# SmartPhone class has more methods than Phone class
iphone.take_photo
# Override method
iphone.change_color('gold', 'polished')
# This does'nt show anything because
# clas instance variable in not accessible from SmartPhone class
SmartPhone.camera?
# Method from Keyboard module
iphone.type('i')
phone.exit
iphone.exit
The following should be the result.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
TYPE : mobile
Camera : NO
Welcome to black Traditional Phone! (instance method)
You changed color to white (instance method)
See you at 2014-02-04 14:24:50 +0100, zzz.... (instance method)
You typed [t] with Traditional Phone.
@@count : 1 ('Class' method with class variable)
Welcome to black iPhone! (instance method)
@@count : 2 ('Class' method with class variable)
You can take photo with smartphone!
-> Photo is saved in iPhone. (Private method)
You changed color to gold (instance method)
Now your phone's surface is polished! (Override instance method)
Camera : (of course dosn't work)
You typed [i] with iPhone.
Good bye! from Traditional Phone (instance method)
Good bye! from iPhone (instance method)
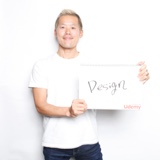