Rubyのチートシート / 式について
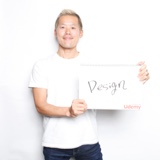
基本だけどRubyっぽいものをまとめてみた。好みもあるだろうけど、良い例、悪い例の比較。Code Schoolがとても分かりやすかったのでお勧め。
ruby
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
# ---------------------------------------------- #
# Expressions
# ---------------------------------------------- #
# 評価に関しての注意
# ---------------------------- #
post = "my post"
#**BAD**#
if post != nil
puts post
end
#**GOOD**# 省略できる
if post
puts post
end
# 下記はTRUEなので注意
# "" -> TRUE
# 0 -> TRUE
# [] -> TRUE
post = ""
puts "#{post}(empty but true)" if post
# 例(これは意図した通りに動かない)
# name.length -> 0でも1でも100でもTRUE
name = ""
unless name.length
puts "Hey! We got an error!"
end
puts "nameは#{name.length}文字だけど評価はTRUE"
# Short-circuit Assignment
# 最初の値がTRUEであればその値を代入する
# ---------------------------- #
result = nil || 1
puts result #-> 1
result = 1 || nil
puts result #-> 1
result = 1 || 2
puts result #-> 1
# 例 (current_sessionがnilならsign_inメソッドを実行)
# def sign_in
# current_session || sign_in
# end
# Conditional Assignment
# 変数が存在しないか、値が'nil/false'でなければセットする
# ---------------------------- #
user ||= 1
puts user #-> 1
user = nil
user ||= 1
puts user #-> 1
user = false
user ||= 1
puts user #-> 1
user = true
user ||= 1
puts user #-> true
# Conditional Return Values
# ifの前に変数を置く(if文はいつも値を返す)
# ---------------------------- #
#**BAD**#
if user
message = "Welcome!"
else
message = "Please sign in."
end
#**GOOD**#
message = if user
"Welcome!"
else
"Please sign in."
end
puts "#{message} (Conditional Return Values)"
# Conditional Return Values
# 代入しなくてもよい
# ---------------------------- #
#**BAD**#
def get_url(user_id)
if user_id
url = "http://myblog.com/posts/#{id}"
else
url = "http://myblog.com"
end
url
end
#**GOOD**#
def get_url(user_id)
if user_id
"http://myblog.com/posts/#{user_id}"
else
"http://myblog.com"
end
end
puts "#{get_url(14)} (Conditional Return Values)"
# Conditional Return Values
# case文も値を返す
# ---------------------------- #
type = "facebook"
#**GOOD**#
base_url = case type
when "twitter"
"http://twitter.com"
when "facebook"
"http://facebook.com"
else
nil
end
#**BETTER**#
base_url = case type
when "twitter" then "http://twitter.com"
when "facebook" then "http://facebook.com"
else nil
end
puts "#{base_url} (Conditional Return Values)"
上のコードを実行するとこうなるはず。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
$ ruby expression.rb
my post
my post
(empty but true)
nameは0文字だけど評価はTRUE
1
1
1
1
1
1
true
Welcome! (Conditional Return Values)
http://myblog.com/posts/14 (Conditional Return Values)
http://facebook.com (Conditional Return Values)
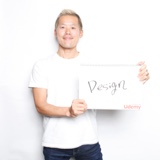